Python Lists: Creation and Access
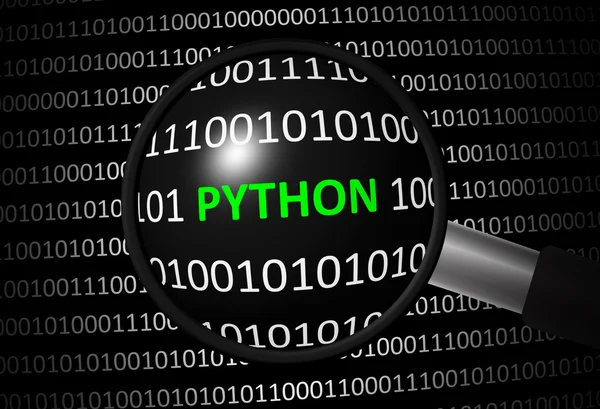
Lists in Python are versatile data structures used to store collections of items. They are ordered, changeable, and allow duplicate values.
**Creating Lists**
Lists are defined using square brackets `[]`, with items separated by commas:
fruits = [“apple”, “banana”, “cherry”]
numbers = [1, 2, 3, 4, 5]
**Accessing List Items**
Items in a list can be accessed using indices. Python uses zero-based indexing:
print(fruits[0]) # Output: “apple”
**Modifying Lists**
Lists are mutable, meaning you can change their content:
fruits[1] = “blueberry”
print(fruits) # Output: [“apple”, “blueberry”, “cherry”]
**List Methods**
Python lists come with several built-in methods:
– `append()`: Adds an item to the end of the list.
– `remove()`: Removes the first occurrence of a specified value.
– `sort()`: Sorts the list in ascending order.
– `reverse()`: Reverses the order of items in the list.
**Example**:
fruits.append(“orange”)
fruits.sort()
print(fruits) # Output: [“apple”, “blueberry”, “cherry”, “orange”]
**Conclusion**
Lists are essential for managing collections of data. They offer flexibility and functionality for various programming tasks.