Python File Handling: Reading and Writing
1 min read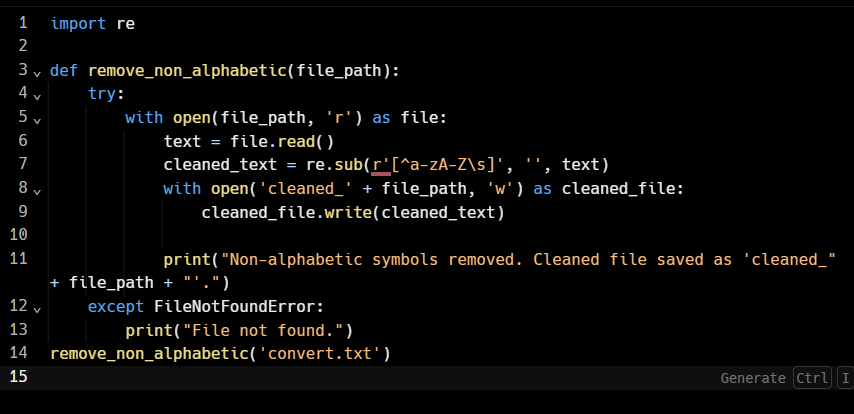
File handling in Python allows you to work with files on your computer. You can read from and write to files using built-in functions.
**Opening Files**
Files are opened using the `open()` function, with modes such as `r` (read), `w` (write), and `a` (append):
file = open(“example.txt”, “w”)
file.write(“Hello, world!”)
file.close()
**Reading Files**
To read from a file, open it in `r` mode and use methods like `read()`, `readline()`, or `readlines()`:
file = open(“example.txt”, “r”)
content = file.read()
print(content)
file.close()
**Example**:
with open(“example.txt”, “w”) as file:
file.write(“Hello, Python!”)
with open(“example.txt”, “r”) as file:
content = file.read()
print(content)
**Conclusion**
File handling is a fundamental skill for reading from and writing to files, essential for many programming tasks.