Python Data Types: Understanding Integers and Floats
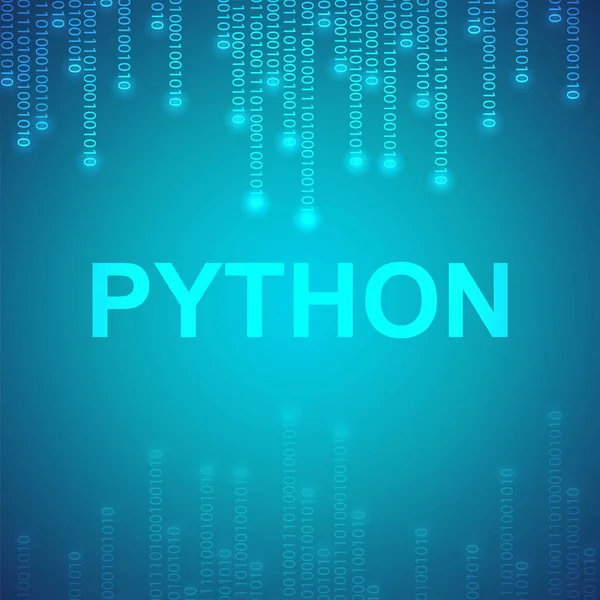
Python supports various data types to handle different kinds of data. Among these, integers and floating-point numbers are two fundamental types.
**Integers**
Integers represent whole numbers without a fractional component. They can be positive or negative.
**Example**:
age = 30 # This is an integer
print(f”Age: {age}”)
**Floating-Point Numbers**
Floats represent real numbers and are used for numbers with a decimal point. They can also be positive or negative.
**Example**:
height = 5.9 # This is a float
print(f”Height: {height}”)
**Type Conversion**
You can convert between integers and floats using built-in functions:
int_value = int(3.14)
float_value = float(10)
**Arithmetic Operations**
You can perform arithmetic operations with these types:
result = age + height
print(f”Combined Result: {result}”)
**Conclusion**
Mastering data types is crucial for manipulating and processing data in Python. Understanding the difference between integers and floats helps you choose the right type for your needs.