Understanding Label Encoding
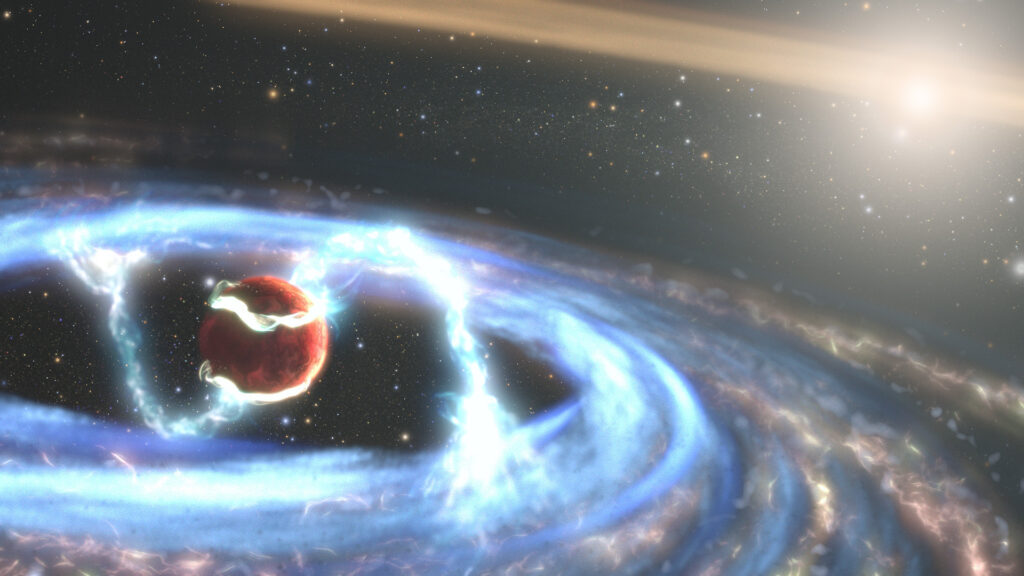
In machine learning, data is often in a form that the computer cannot understand directly. For example, we may have categories like “Pass” and “Fail” in the data, but the model requires numerical inputs. Label encoding is a way to convert these categories into numbers.
Let’s continue with our student example. Imagine we want to create a system that predicts whether students will pass or fail based on their scores. We might label their results as “Pass” or “Fail”:
scores = [30, 60, 55, 45] labels = ['Fail', 'Pass', 'Pass', 'Fail']
Since machine learning algorithms work better with numbers, we convert these labels into numerical values:
encoded_labels = [1 if label == 'Pass' else 0 for label in labels] print(encoded_labels)
Here, the system assigns 1
for “Pass” and 0
for “Fail”. This is called label encoding.
Exoplanet Data: In the exoplanet dataset, the labels are 1
for exoplanets and 2
for stars. Since we are interested in a binary classification (whether an object is an exoplanet or not), we relabel them as 1
for exoplanets and 0
for stars:
categ = {2: 1, 1: 0} train_data.LABEL = [categ[item] for item in train_data.LABEL] test_data.LABEL = [categ[item] for item in test_data.LABEL]
This conversion simplifies the model’s task of distinguishing between exoplanets and stars. Label encoding is a crucial step in transforming categorical data into a format suitable for machine learning.